Algorithmic Trading Python Pdf
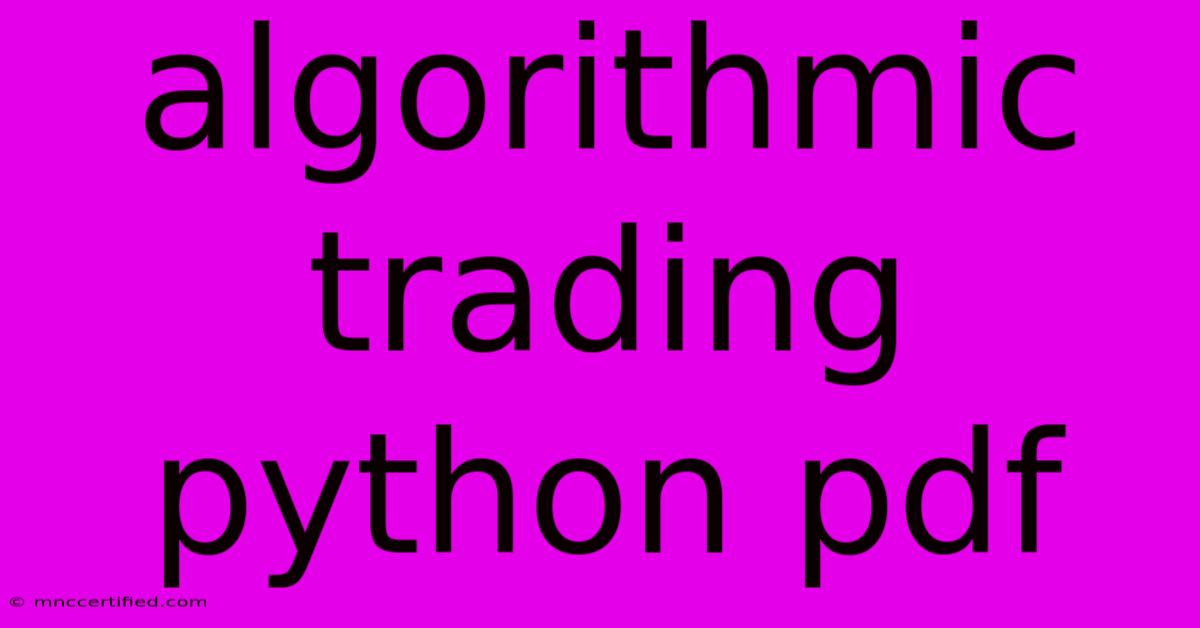
Table of Contents
Algorithmic Trading with Python: A Comprehensive Guide
Algorithmic trading, or algo-trading, is revolutionizing the financial markets. This automated approach leverages sophisticated algorithms to execute trades at optimal prices and speeds, surpassing human capabilities. This guide delves into the world of algorithmic trading using Python, a powerful and versatile programming language well-suited for quantitative finance. While a comprehensive guide in PDF format is beyond the scope of this text-based response (PDF creation requires specific software and formatting tools), this article will equip you with the core knowledge and resources to start your journey.
Why Python for Algorithmic Trading?
Python's dominance in algorithmic trading stems from several key advantages:
- Extensive Libraries: Python boasts powerful libraries like
pandas
(for data manipulation),NumPy
(for numerical computation),Scikit-learn
(for machine learning), andTA-Lib
(for technical analysis), significantly simplifying the development process. - Ease of Use: Python's readability and intuitive syntax make it easier to learn and use than languages like C++ or Java, reducing the time needed to develop and deploy trading strategies.
- Large Community: A vast and active community ensures readily available support, tutorials, and readily available solutions to common problems.
- Integration Capabilities: Python seamlessly integrates with various brokerage APIs, facilitating direct interaction with trading platforms.
Getting Started: Essential Tools and Libraries
Before diving into code, ensure you have the necessary tools installed. This includes:
- Python Installation: Download and install the latest version of Python from .
- Anaconda (Recommended): Anaconda is a user-friendly distribution that bundles Python with essential scientific libraries, simplifying installation and package management. Download it from .
- IDE: Choose a suitable Integrated Development Environment (IDE) like Jupyter Notebook (interactive coding), VS Code (versatile and feature-rich), or PyCharm (powerful professional IDE).
Install the core libraries using pip (or conda within Anaconda):
pip install pandas numpy scikit-learn TA-Lib
Building Your First Algorithmic Trading Strategy
Let's outline a simple moving average crossover strategy. This strategy buys when a short-term moving average crosses above a long-term moving average and sells when the opposite occurs.
This is a simplified example; real-world strategies require more robust risk management and backtesting. Never use real money without thorough backtesting and risk management.
import pandas as pd
import yfinance as yf # For fetching stock data
# Fetch stock data
data = yf.download("AAPL", start="2022-01-01", end="2023-01-01")
# Calculate moving averages
data['SMA_short'] = data['Close'].rolling(window=50).mean()
data['SMA_long'] = data['Close'].rolling(window=200).mean()
# Generate trading signals
data['Signal'] = 0.0
data['Signal'][data['SMA_short'] > data['SMA_long']] = 1.0
data['Position'] = data['Signal'].diff()
# (Further analysis and strategy execution would follow here)
print(data)
This code fetches Apple (AAPL) stock data, calculates 50-day and 200-day Simple Moving Averages (SMAs), and generates buy (1) and sell (-1) signals based on the crossover. Remember, this is a basic illustration. A complete trading system requires sophisticated backtesting, risk management, and order execution mechanisms.
Advanced Topics: Machine Learning and Backtesting
Algorithmic trading extends far beyond simple moving averages. Advanced techniques include:
- Machine Learning: Employing machine learning models (e.g., Support Vector Machines, Neural Networks) to predict price movements and optimize trading strategies.
- Backtesting: Rigorously testing trading strategies on historical data to evaluate their performance and identify potential weaknesses. Libraries like
backtrader
are invaluable for backtesting. - Risk Management: Implementing strategies to mitigate potential losses, including stop-loss orders and position sizing.
- Order Management: Developing robust order placement and execution logic to minimize slippage and maximize efficiency.
Conclusion
This guide provides a foundational understanding of algorithmic trading with Python. Mastering this field requires consistent learning, rigorous testing, and a deep understanding of financial markets. Remember to focus on robust risk management and always prioritize responsible trading practices. While a PDF encompassing all aspects of algorithmic trading is a significant undertaking, this article offers a solid starting point for your learning journey. Remember to consult additional resources and continue learning to stay ahead in this rapidly evolving field.
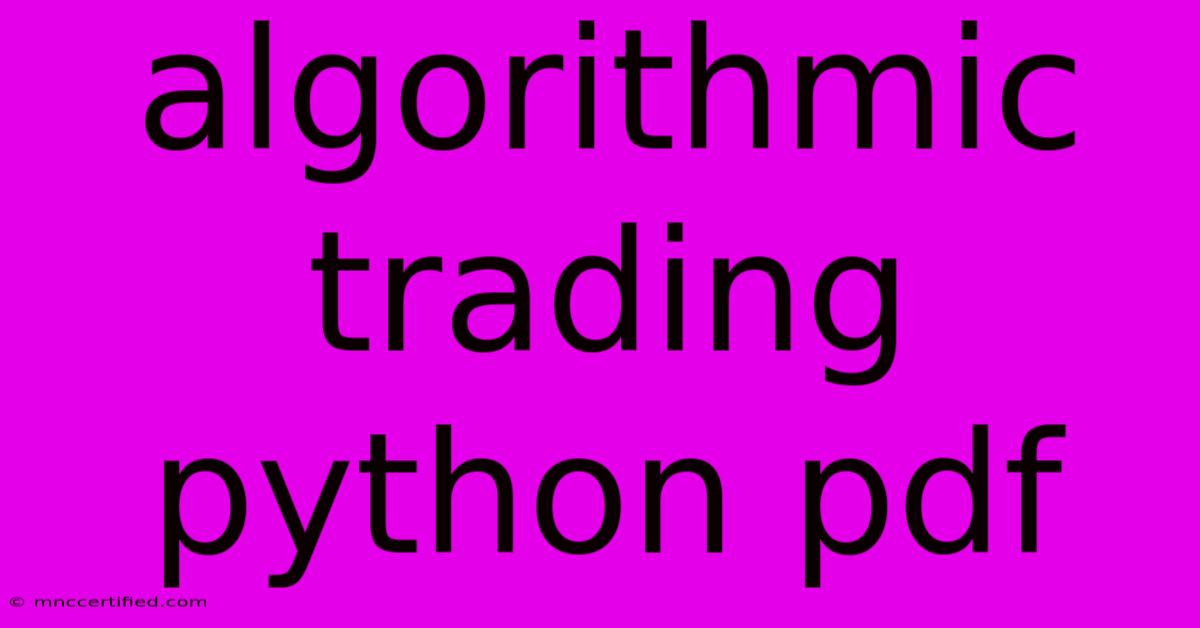
Thank you for visiting our website wich cover about Algorithmic Trading Python Pdf. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Swimming Pool Bonding Diagram
Nov 28, 2024
-
Savannah Bond Onlyfans Videos
Nov 28, 2024
-
Barry Bonds Rookie Card Fleer
Nov 28, 2024
-
Hudson River Trading Interview
Nov 28, 2024
-
Dee Devlins Message For Mc Gregor
Nov 28, 2024